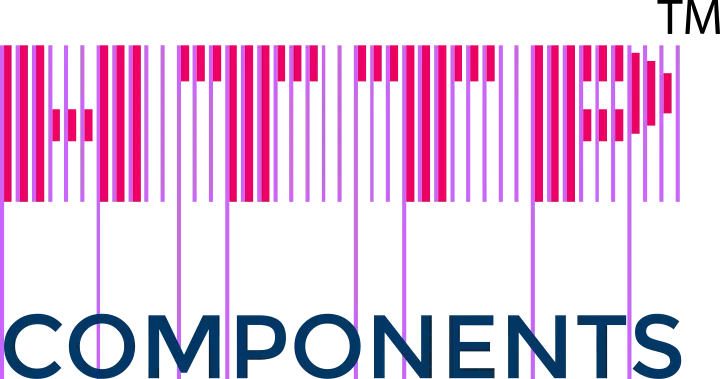
Hypertext Transfer Protocol (HTTP) is probably one of the most important protocols on the Internet today. Web services, microservices, and services on various devices that support the Web are almost all HTTP, and the HTTP protocol has moved from Web browsers to a wider range of usage scenarios.
Although the java.net
package already provides the basic functionality for HTTP access to resources, it is not flexible enough and cannot be customized on a whim. Apache HttpClient 5 is an open source HTTP toolkit that supports the latest HTTP protocol standards and has a rich API and powerful extensions that can be used to build any application that requires HTTP protocol processing applications.
This article introduces some of the most common uses of Apache HttpClient 5, through this article you can quickly get started using HttpClient 5, the main content includes HttpClient 5 Get requests, Post requests, how to submit form parameters, query parameters, JSON data, set the timeout, asynchronous requests, operation Cookie, form login, basic authentication, Digest authentication, and custom HTTP request interceptor.
HttpClient 5 dependencies
Maven
1
2
3
4
5
6
7
8
9
10
11
12
|
<!-- https://mvnrepository.com/artifact/org.apache.httpcomponents.client5/httpclient5 -->
<dependency>
<groupId>org.apache.httpcomponents.client5</groupId>
<artifactId>httpclient5</artifactId>
<version>5.1.3</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.httpcomponents.client5/httpclient5-fluent -->
<dependency>
<groupId>org.apache.httpcomponents.client5</groupId>
<artifactId>httpclient5-fluent</artifactId>
<version>5.1.3</version>
</dependency>
|
Gradle
1
2
|
implementation 'org.apache.httpcomponents.client5:httpclient5:5.1.3'
implementation 'org.apache.httpcomponents.client5:httpclient5-fluent:5.1.3'
|
HttpClient 5 GET request
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
|
package com.wdbyte.httpclient;
import java.io.IOException;
import org.apache.hc.client5.http.classic.methods.HttpGet;
import org.apache.hc.client5.http.impl.classic.CloseableHttpClient;
import org.apache.hc.client5.http.impl.classic.CloseableHttpResponse;
import org.apache.hc.client5.http.impl.classic.HttpClients;
import org.apache.hc.core5.http.HttpEntity;
import org.apache.hc.core5.http.ParseException;
import org.apache.hc.core5.http.io.entity.EntityUtils;
/**
* @author https://www.wdbyte.com
*/
public class HttpClient5Get {
public static void main(String[] args) {
String result = get("http://httpbin.org/get");
System.out.println(result);
}
public static String get(String url) {
String resultContent = null;
HttpGet httpGet = new HttpGet(url);
try (CloseableHttpClient httpclient = HttpClients.createDefault()) {
try (CloseableHttpResponse response = httpclient.execute(httpGet)) {
// Get status code
System.out.println(response.getVersion()); // HTTP/1.1
System.out.println(response.getCode()); // 200
System.out.println(response.getReasonPhrase()); // OK
HttpEntity entity = response.getEntity();
// Get response information
resultContent = EntityUtils.toString(entity);
}
} catch (IOException | ParseException e) {
e.printStackTrace();
}
return resultContent;
}
}
|
Response message.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
HTTP/1.1
200
OK
{
"args": {},
"headers": {
"Accept-Encoding": "gzip, x-gzip, deflate",
"Host": "httpbin.org",
"User-Agent": "Apache-HttpClient/5.1.3 (Java/17)",
"X-Amzn-Trace-Id": "Root=1-62bb1891-5ab5e5376ed960471bf32f17"
},
"origin": "47.251.4.198",
"url": "http://httpbin.org/get"
}
|
HttpClient 5 Fluent GET
Use the Fluent API provided by Apache HttpClient 5 to make it easier to initiate GET requests.
Dependencies.
1
2
3
4
5
6
|
<!-- https://mvnrepository.com/artifact/org.apache.httpcomponents.client5/httpclient5-fluent -->
<dependency>
<groupId>org.apache.httpcomponents.client5</groupId>
<artifactId>httpclient5-fluent</artifactId>
<version>5.1.3</version>
</dependency>
|
Example.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
|
package com.wdbyte.httpclient;
import java.io.IOException;
import org.apache.hc.client5.http.fluent.Request;
import org.apache.hc.client5.http.fluent.Response;
/**
* @author https://www.wdbyte.com
*/
public class HttpClient5GetFluent {
public static void main(String[] args) {
System.out.println(get("http://httpbin.org/get"));
}
public static String get(String url) {
String result = null;
try {
Response response = Request.get(url).execute();
result = response.returnContent().asString();
} catch (IOException e) {
e.printStackTrace();
}
return result;
}
}
|
The output is as follows.
1
2
3
4
5
6
7
8
9
10
11
|
{
"args": {},
"headers": {
"Accept-Encoding": "gzip, x-gzip, deflate",
"Host": "httpbin.org",
"User-Agent": "Apache-HttpClient/5.1.3 (Java/17)",
"X-Amzn-Trace-Id": "Root=1-62bb190e-1ba46a92645843a04c55da32"
},
"origin": "47.251.4.198",
"url": "http://httpbin.org/get"
}
|
HttpClient5 GET Query Parameters
Use the addParameters()
method of the URIBuilder
to construct the parameters of a GET request.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
|
package com.wdbyte.httpclient;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.ArrayList;
import java.util.List;
import org.apache.hc.client5.http.classic.methods.HttpGet;
import org.apache.hc.client5.http.impl.classic.CloseableHttpClient;
import org.apache.hc.client5.http.impl.classic.CloseableHttpResponse;
import org.apache.hc.client5.http.impl.classic.HttpClients;
import org.apache.hc.core5.http.HttpEntity;
import org.apache.hc.core5.http.NameValuePair;
import org.apache.hc.core5.http.ParseException;
import org.apache.hc.core5.http.io.entity.EntityUtils;
import org.apache.hc.core5.http.message.BasicNameValuePair;
import org.apache.hc.core5.net.URIBuilder;
/**
* @author https://www.wdbyte.com
*/
public class HttpClient5GetParams {
public static void main(String[] args) {
String result = get("http://httpbin.org/get");
System.out.println(result);
}
public static String get(String url) {
String resultContent = null;
HttpGet httpGet = new HttpGet(url);
List<NameValuePair> nvps = new ArrayList<>();
// GET Query Parameters
nvps.add(new BasicNameValuePair("username", "wdbyte.com"));
nvps.add(new BasicNameValuePair("password", "secret"));
// Add to the request URL
try {
URI uri = new URIBuilder(new URI(url))
.addParameters(nvps)
.build();
httpGet.setUri(uri);
} catch (URISyntaxException e) {
throw new RuntimeException(e);
}
try (CloseableHttpClient httpclient = HttpClients.createDefault()) {
try (CloseableHttpResponse response = httpclient.execute(httpGet)) {
System.out.println(response.getVersion()); // HTTP/1.1
System.out.println(response.getCode()); // 200
System.out.println(response.getReasonPhrase()); // OK
HttpEntity entity = response.getEntity();
resultContent = EntityUtils.toString(entity);
}
} catch (IOException | ParseException e) {
e.printStackTrace();
}
return resultContent;
}
}
|
The output is as follows.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
{
"args": {
"password": "secret",
"username": "wdbyte.com"
},
"headers": {
"Accept-Encoding": "gzip, x-gzip, deflate",
"Host": "httpbin.org",
"User-Agent": "Apache-HttpClient/5.1.3 (Java/1.8.0_151)",
"X-Amzn-Trace-Id": "Root=1-62ecc660-69d58a226aefb1b6226541ec"
},
"origin": "42.120.75.185",
"url": "http://httpbin.org/get?username=wdbyte.com&password=secret"
}
|
The following is the format of the request response message obtained through network monitoring.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
|
// Request =================
GET /get?username=wdbyte.com&password=secret HTTP/1.1
Accept-Encoding: gzip, x-gzip, deflate
Host: httpbin.org
Connection: keep-alive
User-Agent: Apache-HttpClient/5.1.3 (Java/1.8.0_151)
// Response ===============
HTTP/1.1 200 OK
Date: Fri, 05 Aug 2022 07:27:30 GMT
Content-Type: application/json
Content-Length: 405
Connection: keep-alive
Server: gunicorn/19.9.0
Access-Control-Allow-Origin: *
Access-Control-Allow-Credentials: true
{
"args": {
"password": "secret",
"username": "wdbyte.com"
},
"headers": {
"Accept-Encoding": "gzip, x-gzip, deflate",
"Host": "httpbin.org",
"User-Agent": "Apache-HttpClient/5.1.3 (Java/1.8.0_151)",
"X-Amzn-Trace-Id": "Root=1-62ecc660-69d58a226aefb1b6226541ec"
},
"origin": "42.120.75.185",
"url": "http://httpbin.org/get?username=wdbyte.com&password=secret"
}
|
HttpClient 5 POST Request
The following demonstrates initiating a POST request with form parameters.
Parameters: username=wdbyte.com&password=secret
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
|
package com.wdbyte.httpclient;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.apache.hc.client5.http.classic.methods.HttpPost;
import org.apache.hc.client5.http.entity.UrlEncodedFormEntity;
import org.apache.hc.client5.http.impl.classic.CloseableHttpClient;
import org.apache.hc.client5.http.impl.classic.CloseableHttpResponse;
import org.apache.hc.client5.http.impl.classic.HttpClients;
import org.apache.hc.core5.http.HttpEntity;
import org.apache.hc.core5.http.NameValuePair;
import org.apache.hc.core5.http.ParseException;
import org.apache.hc.core5.http.io.entity.EntityUtils;
import org.apache.hc.core5.http.message.BasicNameValuePair;
/**
* @author https://www.wdbyte.com
*/
public class HttpClient5Post {
public static void main(String[] args) {
String result = post("http://httpbin.org/post");
System.out.println(result);
}
public static String post(String url) {
String result = null;
HttpPost httpPost = new HttpPost(url);
// form parameters.
List<NameValuePair> nvps = new ArrayList<>();
nvps.add(new BasicNameValuePair("username", "wdbyte.com"));
nvps.add(new BasicNameValuePair("password", "secret"));
httpPost.setEntity(new UrlEncodedFormEntity(nvps));
try (CloseableHttpClient httpclient = HttpClients.createDefault()) {
try (CloseableHttpResponse response = httpclient.execute(httpPost)) {
System.out.println(response.getVersion()); // HTTP/1.1
System.out.println(response.getCode()); // 200
System.out.println(response.getReasonPhrase()); // OK
HttpEntity entity = response.getEntity();
result = EntityUtils.toString(entity);
// Ensure that the stream is fully consumed
EntityUtils.consume(entity);
}
} catch (IOException | ParseException e) {
e.printStackTrace();
}
return result;
}
}
|
The output is as follows.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
HTTP/1.1
200
OK
{
"args": {},
"data": "",
"files": {},
"form": {
"password": "secret",
"username": "wdbyte.com"
},
"headers": {
"Accept-Encoding": "gzip, x-gzip, deflate",
"Content-Length": "35",
"Content-Type": "application/x-www-form-urlencoded; charset=ISO-8859-1",
"Host": "httpbin.org",
"User-Agent": "Apache-HttpClient/5.1.3 (Java/17)",
"X-Amzn-Trace-Id": "Root=1-62bb1ac8-489b2100728c81d70797a482"
},
"json": null,
"origin": "183.128.136.89",
"url": "http://httpbin.org/post"
}
|
Below is the request information obtained through network monitoring.
1
2
3
4
5
6
7
8
9
|
POST /post HTTP/1.1
Accept-Encoding: gzip, x-gzip, deflate
Content-Length: 35
Content-Type: application/x-www-form-urlencoded; charset=ISO-8859-1
Host: httpbin.org
Connection: keep-alive
User-Agent: Apache-HttpClient/5.1.3 (Java/17)
username=wdbyte.com&password=secret
|
HttpClient 5 Fluent POST
Using the Fluent API provided by Apache HttpClient 5 makes it easier to initiate POST requests.
As well, send a simple form parameter: username=wdbyte.com&password=secret
.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
|
package com.wdbyte.httpclient;
import java.io.IOException;
import org.apache.hc.client5.http.fluent.Request;
import org.apache.hc.core5.http.message.BasicNameValuePair;
/**
* @author https://www.wdbyte.com
*/
public class HttpClient5PostFluent {
public static void main(String[] args) {
String result = post("http://httpbin.org/post");
System.out.println(result);
}
public static String post(String url) {
String result = null;
Request request = Request.post(url);
// POST Body
request.bodyForm(
new BasicNameValuePair("username", "wdbyte.com"),
new BasicNameValuePair("password", "secret"));
try {
result = request.execute().returnContent().asString();
} catch (IOException e) {
e.printStackTrace();
}
return result;
}
}
|
The output is as follows.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
{
"args": {},
"data": "",
"files": {},
"form": {
"password": "secret",
"username": "wdbyte.com"
},
"headers": {
"Accept-Encoding": "gzip, x-gzip, deflate",
"Content-Length": "35",
"Content-Type": "application/x-www-form-urlencoded; charset=ISO-8859-1",
"Host": "httpbin.org",
"User-Agent": "Apache-HttpClient/5.1.3 (Java/17)",
"X-Amzn-Trace-Id": "Root=1-62bb1c8a-7aee8c004f06919f31a2b533"
},
"json": null,
"origin": "183.128.136.89",
"url": "http://httpbin.org/post"
}
|
HttpClient5 POST JSON data
Use the StringEntity
class to store JSON data.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
|
package com.wdbyte.httpclient;
import java.io.IOException;
import org.apache.hc.client5.http.classic.methods.HttpPost;
import org.apache.hc.client5.http.impl.classic.CloseableHttpClient;
import org.apache.hc.client5.http.impl.classic.CloseableHttpResponse;
import org.apache.hc.client5.http.impl.classic.HttpClients;
import org.apache.hc.core5.http.ParseException;
import org.apache.hc.core5.http.io.entity.EntityUtils;
import org.apache.hc.core5.http.io.entity.StringEntity;
/**
* @author https://www.wdbyte.com
*/
public class HttpClient5PostWithJson {
public static void main(String[] args) {
String json = "{"
+ " \"password\": \"secret\","
+ " \"username\": \"wdbyte.com\""
+ "}";
String result = post("http://httpbin.org/post", json);
System.out.println(result);
}
public static String post(String url, String jsonBody) {
String result = null;
HttpPost httpPost = new HttpPost(url);
httpPost.setEntity(new StringEntity(jsonBody, ContentType.APPLICATION_JSON));
try (CloseableHttpClient httpclient = HttpClients.createDefault()) {
try (CloseableHttpResponse response = httpclient.execute(httpPost)) {
result = EntityUtils.toString(response.getEntity());
}
} catch (IOException | ParseException e) {
e.printStackTrace();
}
return result;
}
}
|
The output is as follows.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
{
"args": {},
"data": "{ \"password\": \"secret\", \"username\": \"wdbyte.com\"}",
"files": {},
"form": {},
"headers": {
"Accept-Encoding": "gzip, x-gzip, deflate",
"Content-Length": "55",
"Content-Type": "text/plain; charset=ISO-8859-1",
"Host": "httpbin.org",
"User-Agent": "Apache-HttpClient/5.1.3 (Java/17)",
"X-Amzn-Trace-Id": "Root=1-62bb1dbb-5a963c1d798b06be3ee1a15e"
},
"json": {
"password": "secret",
"username": "wdbyte.com"
},
"origin": "183.128.136.89",
"url": "http://httpbin.org/post"
}
|
The following is the request and response information obtained through network monitoring.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
|
// Request==================
POST /post HTTP/1.1
Accept-Encoding: gzip, x-gzip, deflate
Content-Length: 55
Content-Type: application/json; charset=UTF-8
Host: httpbin.org
Connection: keep-alive
User-Agent: Apache-HttpClient/5.1.3 (Java/17)
{ "password": "secret", "username": "wdbyte.com"}
// Response==================
HTTP/1.1 200 OK
Date: Tue, 28 Jun 2022 15:30:17 GMT
Content-Type: application/json
Content-Length: 573
Connection: keep-alive
Server: gunicorn/19.9.0
Access-Control-Allow-Origin: *
Access-Control-Allow-Credentials: true
{
"args": {},
"data": "{ \"password\": \"secret\", \"username\": \"wdbyte.com\"}",
"files": {},
"form": {},
"headers": {
"Accept-Encoding": "gzip, x-gzip, deflate",
"Content-Length": "55",
"Content-Type": "application/json; charset=UTF-8",
"Host": "httpbin.org",
"User-Agent": "Apache-HttpClient/5.1.3 (Java/17)",
"X-Amzn-Trace-Id": "Root=1-62bb1e89-64db55730a0361c720232ccd"
},
"json": {
"password": "secret",
"username": "wdbyte.com"
},
"origin": "183.128.136.89",
"url": "http://httpbin.org/post"
}
|
HttpClient 5 Setting the Timeout
Use the RequestConfig
object to configure the timeout.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
|
package com.wdbyte.httpclient;
import java.io.IOException;
import org.apache.hc.client5.http.classic.methods.HttpGet;
import org.apache.hc.client5.http.config.RequestConfig;
import org.apache.hc.client5.http.impl.classic.CloseableHttpClient;
import org.apache.hc.client5.http.impl.classic.CloseableHttpResponse;
import org.apache.hc.client5.http.impl.classic.HttpClients;
import org.apache.hc.core5.http.HttpEntity;
import org.apache.hc.core5.http.ParseException;
import org.apache.hc.core5.http.io.entity.EntityUtils;
import org.apache.hc.core5.util.Timeout;
/**
* @author https://www.wdbyte.com
*/
public class HttpClient5GetWithTimeout {
public static void main(String[] args) {
String result = get("http://httpbin.org/get");
System.out.println(result);
}
public static String get(String url) {
String resultContent = null;
// Set timeout time
RequestConfig config = RequestConfig.custom()
.setConnectTimeout(Timeout.ofMilliseconds(5000L))
.setConnectionRequestTimeout(Timeout.ofMilliseconds(5000L))
.setResponseTimeout(Timeout.ofMilliseconds(5000L))
.build();
// Request level timeout
HttpGet httpGet = new HttpGet(url);
//httpGet.setConfig(config);
//try (CloseableHttpClient httpclient = HttpClients.createDefault()) {
// Client-level timeouts
try (CloseableHttpClient httpclient = HttpClients.custom().setDefaultRequestConfig(config).build()) {
try (CloseableHttpResponse response = httpclient.execute(httpGet)) {
System.out.println(response.getVersion()); // HTTP/1.1
System.out.println(response.getCode()); // 200
System.out.println(response.getReasonPhrase()); // OK
HttpEntity entity = response.getEntity();
resultContent = EntityUtils.toString(entity);
}
} catch (IOException | ParseException e) {
e.printStackTrace();
}
return resultContent;
}
}
|
HttpClient 5 asynchronous requests
The following demonstrates the three HttpClient 5 asynchronous request methods.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
|
package com.wdbyte.httpclient;
import java.io.IOException;
import java.nio.CharBuffer;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
import org.apache.hc.client5.http.async.methods.AbstractCharResponseConsumer;
import org.apache.hc.client5.http.async.methods.SimpleHttpRequest;
import org.apache.hc.client5.http.async.methods.SimpleHttpRequests;
import org.apache.hc.client5.http.async.methods.SimpleHttpResponse;
import org.apache.hc.client5.http.impl.async.CloseableHttpAsyncClient;
import org.apache.hc.client5.http.impl.async.HttpAsyncClients;
import org.apache.hc.core5.concurrent.FutureCallback;
import org.apache.hc.core5.http.ContentType;
import org.apache.hc.core5.http.HttpException;
import org.apache.hc.core5.http.HttpResponse;
import org.apache.hc.core5.http.nio.AsyncRequestProducer;
import org.apache.hc.core5.http.nio.support.AsyncRequestBuilder;
/**
* HttpClient 5 异æ¥è¯·æ±‚
* @author https://www.wdbyte.com
* @date 2022/06/25
*/
public class HttpClient5Async {
public static void main(String[] args) {
getAsync1("http://httpbin.org/get");
getAsync2("http://httpbin.org/get");
getAsync3("http://httpbin.org/get");
}
/**
* Asynchronous requests
*
* @param url
* @return
*/
public static String getAsync1(String url) {
try (CloseableHttpAsyncClient httpclient = HttpAsyncClients.createDefault()) {
// start http clinet
httpclient.start();
// Execution of requests
SimpleHttpRequest request1 = SimpleHttpRequests.get(url);
Future<SimpleHttpResponse> future = httpclient.execute(request1, null);
// Wait until the return is complete
SimpleHttpResponse response1 = future.get();
System.out.println("getAsync1:" + request1.getRequestUri() + "->" + response1.getCode());
} catch (IOException | ExecutionException | InterruptedException e) {
throw new RuntimeException(e);
}
return null;
}
/**
* Asynchronous requests, callbacks based on response
*
* @param url
* @return
*/
public static String getAsync2(String url) {
try (CloseableHttpAsyncClient httpclient = HttpAsyncClients.createDefault()) {
// start http clinet
httpclient.start();
CountDownLatch latch = new CountDownLatch(1);
SimpleHttpRequest request = SimpleHttpRequests.get(url);
httpclient.execute(request, new FutureCallback<SimpleHttpResponse>() {
@Override
public void completed(SimpleHttpResponse response2) {
latch.countDown();
System.out.println("getAsync2:" + request.getRequestUri() + "->" + response2.getCode());
}
@Override
public void failed(Exception ex) {
latch.countDown();
System.out.println("getAsync2:" + request.getRequestUri() + "->" + ex);
}
@Override
public void cancelled() {
latch.countDown();
System.out.println("getAsync2:" + request.getRequestUri() + " cancelled");
}
});
latch.await();
} catch (IOException | InterruptedException e) {
throw new RuntimeException(e);
}
return null;
}
/**
* Asynchronous requests, do something about the response stream
*
* @param url
* @return
*/
public static String getAsync3(String url) {
try (CloseableHttpAsyncClient httpclient = HttpAsyncClients.createDefault()) {
// start http clinet
httpclient.start();
SimpleHttpRequest request = SimpleHttpRequests.get(url);
CountDownLatch latch = new CountDownLatch(1);
AsyncRequestProducer producer = AsyncRequestBuilder.get("http://httpbin.org/get").build();
AbstractCharResponseConsumer<HttpResponse> consumer3 = new AbstractCharResponseConsumer<HttpResponse>() {
HttpResponse response;
@Override
protected void start(HttpResponse response, ContentType contentType) throws HttpException, IOException {
System.out.println("getAsync3: Start response....");
this.response = response;
}
@Override
protected int capacityIncrement() {
return Integer.MAX_VALUE;
}
@Override
protected void data(CharBuffer data, boolean endOfStream) throws IOException {
System.out.println("getAsync3: Data received....");
// Do something useful
}
@Override
protected HttpResponse buildResult() throws IOException {
System.out.println("getAsync3: Receiving completed...");
return response;
}
@Override
public void releaseResources() {
}
};
httpclient.execute(producer, consumer3, new FutureCallback<HttpResponse>() {
@Override
public void completed(HttpResponse response) {
latch.countDown();
System.out.println("getAsync3: "+request.getRequestUri() + "->" + response.getCode());
}
@Override
public void failed(Exception ex) {
latch.countDown();
System.out.println("getAsync3: "+request.getRequestUri() + "->" + ex);
}
@Override
public void cancelled() {
latch.countDown();
System.out.println("getAsync3: "+request.getRequestUri() + " cancelled");
}
});
latch.await();
} catch (IOException | InterruptedException e) {
throw new RuntimeException(e);
}
return null;
}
}
|
The output is as follows.
1
2
3
4
5
6
7
8
|
getAsync1:/get->200
getAsync2:/get->200
getAsync3: Start response....
getAsync3: Data received....
getAsync3: Data received....
getAsync3: Data received....
getAsync3: Receiving completed...
getAsync3: /get->200
|
HttpClient 5 Get Cookie
The response to the request http://httpbin.org/cookies/set/cookieName/www.wdbyte.com
will have a cookie message with the name cookieName
and the value www.wdbyte.com
, which we use as a test.
Postman request test, you can see the response cookie information.
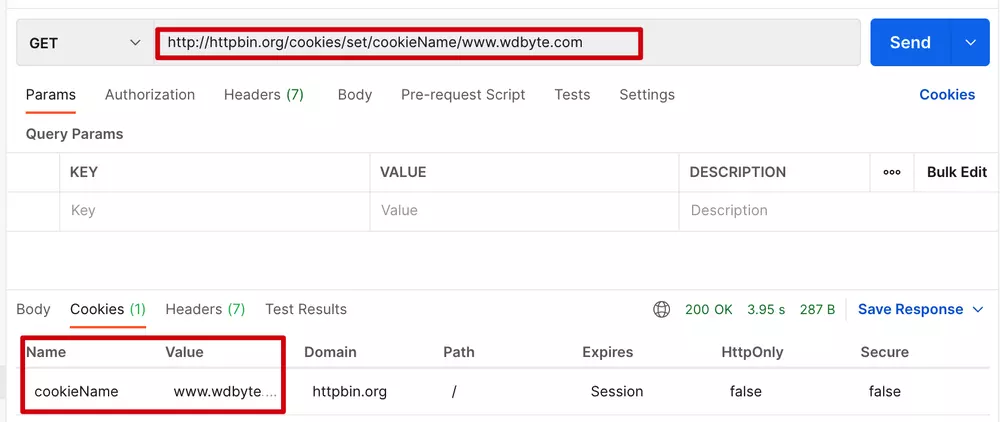
The following Java code is written to test the request.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
|
package com.wdbyte.httpclient;
import java.util.List;
import org.apache.hc.client5.http.classic.methods.HttpGet;
import org.apache.hc.client5.http.cookie.BasicCookieStore;
import org.apache.hc.client5.http.cookie.Cookie;
import org.apache.hc.client5.http.cookie.CookieStore;
import org.apache.hc.client5.http.impl.classic.CloseableHttpClient;
import org.apache.hc.client5.http.impl.classic.CloseableHttpResponse;
import org.apache.hc.client5.http.impl.classic.HttpClients;
import org.apache.hc.client5.http.impl.cookie.BasicClientCookie;
import org.apache.hc.client5.http.protocol.HttpClientContext;
import org.apache.hc.core5.http.io.entity.EntityUtils;
/**
* This example demonstrates the use of native HTTP context filling, custom properties.
*/
public class HttpClient5WithCookie {
public static void main(final String[] args) throws Exception {
try (final CloseableHttpClient httpclient = HttpClients.createDefault()) {
// Create a local cookie store
final CookieStore cookieStore = new BasicCookieStore();
// BasicClientCookie clientCookie = new BasicClientCookie("name", "www.wdbyte.com");
// clientCookie.setDomain("http://httpbin.org/cookies");
// Expiration time
// clientCookie.setExpiryDate(new Date());
// addCookie
// cookieStore.addCookie(clientCookie);
// Create local HttpClientContext
final HttpClientContext localContext = HttpClientContext.create();
// Bind the cookieStore to the localContext
localContext.setCookieStore(cookieStore);
final HttpGet httpget = new HttpGet("http://httpbin.org/cookies/set/cookieName/www.wdbyte.com");
System.out.println("Execute request " + httpget.getMethod() + " " + httpget.getUri());
// Get Cookie information.
try (final CloseableHttpResponse response = httpclient.execute(httpget, localContext)) {
System.out.println("----------------------------------------");
System.out.println(response.getCode() + " " + response.getReasonPhrase());
final List<Cookie> cookies = cookieStore.getCookies();
for (int i = 0; i < cookies.size(); i++) {
System.out.println("Local cookie: " + cookies.get(i));
}
EntityUtils.consume(response.getEntity());
}
}
}
}
|
The output is as follows.
1
2
3
4
|
Execute request GET http://httpbin.org/cookies/set/cookieName/www.wdbyte.com
----------------------------------------
200 OK
Local cookie: [name: cookieName; value: www.wdbyte.com; domain: httpbin.org; path: /; expiry: null]
|
HttpClient 5 Read File Content Request
Prepare a file params.json in JSON content format.
1
|
{"name":"www.wdbyte.com"}
|
Read this file as a request parameter to initiate the request.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
|
package com.wdbyte.httpclient;
import java.io.File;
import java.io.FileInputStream;
import org.apache.hc.client5.http.classic.methods.HttpPost;
import org.apache.hc.client5.http.impl.classic.CloseableHttpClient;
import org.apache.hc.client5.http.impl.classic.CloseableHttpResponse;
import org.apache.hc.client5.http.impl.classic.HttpClients;
import org.apache.hc.core5.http.ContentType;
import org.apache.hc.core5.http.io.entity.EntityUtils;
import org.apache.hc.core5.http.io.entity.FileEntity;
import org.apache.hc.core5.http.io.entity.InputStreamEntity;
/**
* Load the data stream as a POST request body
*/
public class HttpClient5ChunkEncodedPost {
public static void main(final String[] args) throws Exception {
String params = "/Users/darcy/params.json";
try (final CloseableHttpClient httpclient = HttpClients.createDefault()) {
final HttpPost httppost = new HttpPost("http://httpbin.org/post");
final InputStreamEntity reqEntity = new InputStreamEntity(new FileInputStream(params), -1,
ContentType.APPLICATION_JSON);
// You can also use the FileEntity
// FileEntity reqEntity = new FileEntity(new File(params), ContentType.APPLICATION_JSON);
httppost.setEntity(reqEntity);
System.out.println("Execute request " + httppost.getMethod() + " " + httppost.getUri());
try (final CloseableHttpResponse response = httpclient.execute(httppost)) {
System.out.println("----------------------------------------");
System.out.println(response.getCode() + " " + response.getReasonPhrase());
System.out.println(EntityUtils.toString(response.getEntity()));
}
}
}
}
|
The output is as follows.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
Execute request POST http://httpbin.org/post
----------------------------------------
200 OK
{
"args": {},
"data": "{\"name\":\"www.wdbyte.com\"}\n",
"files": {},
"form": {},
"headers": {
"Accept-Encoding": "gzip, x-gzip, deflate",
"Content-Length": "26",
"Content-Type": "application/json; charset=UTF-8",
"Host": "httpbin.org",
"User-Agent": "Apache-HttpClient/5.1.3 (Java/1.8.0_151)",
"X-Amzn-Trace-Id": "Root=1-62ee4d95-1f956d4303cea09c52694c86"
},
"json": {
"name": "www.wdbyte.com"
},
"origin": "42.120.74.238",
"url": "http://httpbin.org/post"
}
|
Form login can be understood as the process of initiating a request with authentication information and then getting a cookie in response. Of course this does not only apply to form login, but can also be a simple request with form information.
I should have used a POST request to send the form parameters for testing, but there is no corresponding interface for testing in httpbin.org, so I switched to a GET request here.
Example code.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
|
package com.wdbyte.httpclient;
import java.util.ArrayList;
import java.util.List;
import org.apache.hc.client5.http.classic.methods.HttpGet;
import org.apache.hc.client5.http.cookie.BasicCookieStore;
import org.apache.hc.client5.http.cookie.Cookie;
import org.apache.hc.client5.http.entity.UrlEncodedFormEntity;
import org.apache.hc.client5.http.impl.classic.CloseableHttpClient;
import org.apache.hc.client5.http.impl.classic.CloseableHttpResponse;
import org.apache.hc.client5.http.impl.classic.HttpClients;
import org.apache.hc.core5.http.HttpEntity;
import org.apache.hc.core5.http.NameValuePair;
import org.apache.hc.core5.http.io.entity.EntityUtils;
import org.apache.hc.core5.http.message.BasicNameValuePair;
/**
* Demo of form-based login
*
* @author https://www.wdbyte.com
*/
public class HttpClient5FormLogin {
public static void main(final String[] args) throws Exception {
final BasicCookieStore cookieStore = new BasicCookieStore();
try (final CloseableHttpClient httpclient = HttpClients.custom()
.setDefaultCookieStore(cookieStore)
.build()) {
// I should have used a POST request to send the form parameters, but there is no corresponding interface in httpbin.org for testing, so I switched to a GET request here
// HttpPost httpPost = new HttpPost("http://httpbin.org/cookies/set/username/wdbyte.com");
HttpGet httpPost = new HttpGet("http://httpbin.org/cookies/set/username/wdbyte.com");
// POST Form
List<NameValuePair> nvps = new ArrayList<>();
nvps.add(new BasicNameValuePair("username", "wdbyte.com"));
nvps.add(new BasicNameValuePair("password", "secret"));
httpPost.setEntity(new UrlEncodedFormEntity(nvps));
try (final CloseableHttpResponse response2 = httpclient.execute(httpPost)) {
final HttpEntity entity = response2.getEntity();
System.out.println("Login form get: " + response2.getCode() + " " + response2.getReasonPhrase());
System.out.println("Current response information "+EntityUtils.toString(entity));;
System.out.println("Post Login Cookie:");
final List<Cookie> cookies = cookieStore.getCookies();
if (cookies.isEmpty()) {
System.out.println("None");
} else {
for (int i = 0; i < cookies.size(); i++) {
System.out.println("- " + cookies.get(i));
}
}
}
}
}
}
|
The output is as follows.
1
2
3
4
5
6
7
8
9
|
Login form get: 200 OK
Current response information {
"cookies": {
"username": "wdbyte.com"
}
}
Post Login Cookie:
- [name: username; value: wdbyte.com; domain: httpbin.org; path: /; expiry: null]
|
HttpClient 5 Basic Authorization
HTTP Basic Authorization is a relatively simple implementation of authentication, the main process is as follows
- request an HTTP interface that requires basic authentication, but does not carry authentication information.
- a 401 status code is responded and the WWW-Authenticate in the response header indicates the need for basic authorization.
- The user splices the required authentication information with a colon, then base64 encodes it, and then splices Basic at the beginning of the resulting string into the request header Authorization.
- The authentication is successful and the response is successful.
You can test the basic authentication by opening the following URL in your browser.
http://httpbin.org/basic-auth/admin/123456
The way it is implemented in Apache HttpClient 5.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
|
package com.wdbyte.httpclient;
import org.apache.hc.client5.http.auth.AuthScope;
import org.apache.hc.client5.http.auth.UsernamePasswordCredentials;
import org.apache.hc.client5.http.classic.methods.HttpGet;
import org.apache.hc.client5.http.impl.auth.BasicCredentialsProvider;
import org.apache.hc.client5.http.impl.classic.CloseableHttpClient;
import org.apache.hc.client5.http.impl.classic.CloseableHttpResponse;
import org.apache.hc.client5.http.impl.classic.HttpClients;
import org.apache.hc.core5.http.io.entity.EntityUtils;
/**
* A simple example that uses HttpClient to perform HTTP requests;
* A target site that requires user authentication.
*/
public class HttpClient5BasicAuthentication {
public static void main(final String[] args) throws Exception {
final BasicCredentialsProvider credsProvider = new BasicCredentialsProvider();
credsProvider.setCredentials(
new AuthScope("httpbin.org", 80),
new UsernamePasswordCredentials("admin", "123456".toCharArray()));
try (final CloseableHttpClient httpclient = HttpClients.custom()
.setDefaultCredentialsProvider(credsProvider)
.build()) {
final HttpGet httpget = new HttpGet("http://httpbin.org/basic-auth/admin/123456");
System.out.println("Execute request " + httpget.getMethod() + " " + httpget.getUri());
try (final CloseableHttpResponse response = httpclient.execute(httpget)) {
System.out.println("----------------------------------------");
System.out.println(response.getCode() + " " + response.getReasonPhrase());
System.out.println(EntityUtils.toString(response.getEntity()));
}
}
}
}
|
The output results are as follows.
1
2
3
4
5
6
7
|
Execute request GET http://httpbin.org/basic-auth/user/passwd
----------------------------------------
200 OK
{
"authenticated": true,
"user": "user"
}
|
The complete HTTP request and response process can be seen through network monitoring.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
|
// Req ==================
GET /basic-auth/user/passwd HTTP/1.1
Accept-Encoding: gzip, x-gzip, deflate
Host: httpbin.org
Connection: keep-alive
User-Agent: Apache-HttpClient/5.1.3 (Java/1.8.0_151)
// Resp=============
HTTP/1.1 401 UNAUTHORIZED
Date: Sat, 06 Aug 2022 08:25:33 GMT
Content-Length: 0
Connection: keep-alive
Server: gunicorn/19.9.0
WWW-Authenticate: Basic realm="Fake Realm"
Access-Control-Allow-Origin: *
Access-Control-Allow-Credentials: true
// Req ==================
GET /basic-auth/user/passwd HTTP/1.1
Host: httpbin.org
Connection: keep-alive
User-Agent: Apache-HttpClient/5.1.3 (Java/1.8.0_151)
Authorization: Basic dXNlcjpwYXNzd2Q=
// Resp=============
HTTP/1.1 200 OK
Date: Sat, 06 Aug 2022 08:25:33 GMT
Content-Type: application/json
Content-Length: 47
Connection: keep-alive
Server: gunicorn/19.9.0
Access-Control-Allow-Origin: *
Access-Control-Allow-Credentials: true
{
"authenticated": true,
"user": "user"
}
|
HttpClient 5 Digest Authorization
The shortcomings of HTTP Basic Authorization are obvious, the password is transmitted in plaintext there is a certain security risk, Digest Authorization authentication method to solve the problem of plaintext transmission, here not to introduce Digest related content, through a simple diagram to illustrate the flow of Digest authentication method.
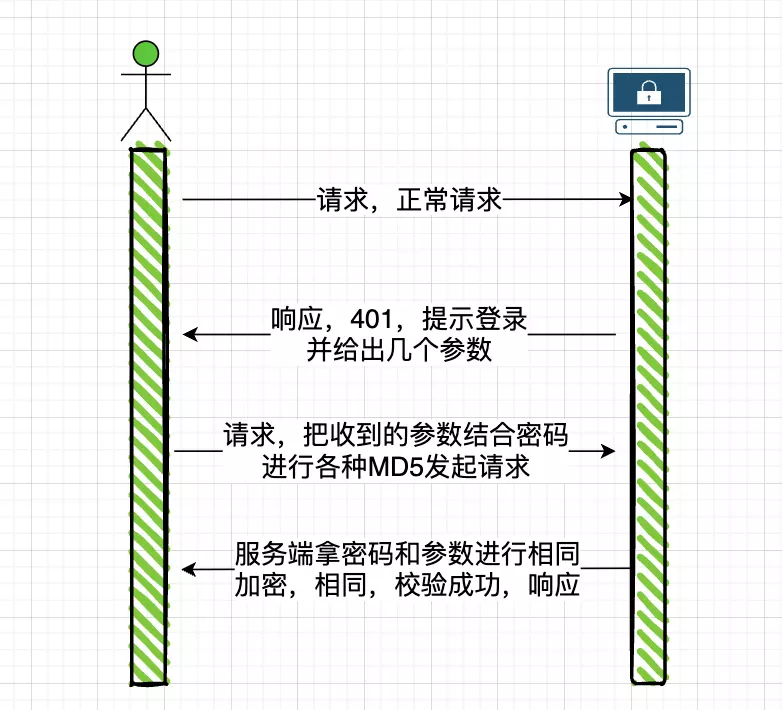
Here is the code demo.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
|
package com.wdbyte.httpclient;
import org.apache.hc.client5.http.auth.AuthExchange;
import org.apache.hc.client5.http.auth.AuthScheme;
import org.apache.hc.client5.http.auth.AuthScope;
import org.apache.hc.client5.http.auth.UsernamePasswordCredentials;
import org.apache.hc.client5.http.classic.methods.HttpGet;
import org.apache.hc.client5.http.impl.auth.BasicCredentialsProvider;
import org.apache.hc.client5.http.impl.auth.DigestScheme;
import org.apache.hc.client5.http.impl.classic.CloseableHttpClient;
import org.apache.hc.client5.http.impl.classic.CloseableHttpResponse;
import org.apache.hc.client5.http.impl.classic.HttpClients;
import org.apache.hc.client5.http.protocol.HttpClientContext;
import org.apache.hc.core5.http.HttpHost;
import org.apache.hc.core5.http.io.entity.EntityUtils;
/**
*
* Example of how HttpClient validates multiple requests
*/
public class HttpClient5PreemptiveDigestAuthentication {
public static void main(final String[] args) throws Exception {
try (final CloseableHttpClient httpclient = HttpClients.createDefault()) {
final HttpHost target = new HttpHost("http", "httpbin.org", 80);
final HttpClientContext localContext = HttpClientContext.create();
final BasicCredentialsProvider credentialsProvider = new BasicCredentialsProvider();
credentialsProvider.setCredentials(
new AuthScope(target),
new UsernamePasswordCredentials("admin", "123456".toCharArray()));
localContext.setCredentialsProvider(credentialsProvider);
final HttpGet httpget = new HttpGet("http://httpbin.org/digest-auth/auth/admin/123456");
System.out.println("Execute request " + httpget.getMethod() + " " + httpget.getUri());
for (int i = 0; i < 2; i++) {
try (final CloseableHttpResponse response = httpclient.execute(target, httpget, localContext)) {
System.out.println("----------------------------------------");
System.out.println(response.getCode() + " " + response.getReasonPhrase());
EntityUtils.consume(response.getEntity());
final AuthExchange authExchange = localContext.getAuthExchange(target);
if (authExchange != null) {
final AuthScheme authScheme = authExchange.getAuthScheme();
if (authScheme instanceof DigestScheme) {
final DigestScheme digestScheme = (DigestScheme) authScheme;
System.out.println("Nonce: " + digestScheme.getNonce() +
"; count: " + digestScheme.getNounceCount());
}
}
}
}
}
}
}
|
The network monitoring tool clearly shows the flow of the 2 requests. In the last request, the authentication information is shared directly without the re-authentication process again.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
|
// 1. Request
GET /digest-auth/auth/admin/123456 HTTP/1.1
Accept-Encoding: gzip, x-gzip, deflate
Host: httpbin.org
Connection: keep-alive
User-Agent: Apache-HttpClient/5.1.3 (Java/1.8.0_151)
// 2. Details, prompts for authentication, gives parameters
HTTP/1.1 401 UNAUTHORIZED
Date: Fri, 12 Aug 2022 07:11:06 GMT
Content-Type: text/html; charset=utf-8
Content-Length: 0
Connection: keep-alive
Server: gunicorn/19.9.0
WWW-Authenticate: Digest realm="me@kennethreitz.com", nonce="8dc5e7974a86a6fcc3cf73230b0c4a93", qop="auth", opaque="64b7f68b386c3acc38131f7472aa2079", algorithm=MD5, stale=FALSE
Set-Cookie: stale_after=never; Path=/
Set-Cookie: fake=fake_value; Path=/
Access-Control-Allow-Origin: *
Access-Control-Allow-Credentials: true
// 3. Parameter + password Encrypt and request again
GET /digest-auth/auth/admin/123456 HTTP/1.1
Host: httpbin.org
Connection: keep-alive
User-Agent: Apache-HttpClient/5.1.3 (Java/1.8.0_151)
Cookie: fake=fake_value; stale_after=never
Authorization: Digest username="admin", realm="me@kennethreitz.com", nonce="8dc5e7974a86a6fcc3cf73230b0c4a93", uri="/digest-auth/auth/admin/123456", response="7c6726f8ac54c1ba28e19c71b2fc7338", qop=auth, nc=00000001, cnonce="2fa61501d47a9d39", algorithm=MD5, opaque="64b7f68b386c3acc38131f7472aa2079"
// 4. Authentication successful, response
HTTP/1.1 200 OK
Date: Fri, 12 Aug 2022 07:11:08 GMT
Content-Type: application/json
Content-Length: 48
Connection: keep-alive
Server: gunicorn/19.9.0
Set-Cookie: fake=fake_value; Path=/
Set-Cookie: stale_after=never; Path=/
Access-Control-Allow-Origin: *
Access-Control-Allow-Credentials: true
{
"authenticated": true,
"user": "admin"
}
// 5. The request was made again and the login status was shared.
GET /digest-auth/auth/admin/123456 HTTP/1.1
Accept-Encoding: gzip, x-gzip, deflate
Host: httpbin.org
Connection: keep-alive
User-Agent: Apache-HttpClient/5.1.3 (Java/1.8.0_151)
Cookie: fake=fake_value; stale_after=never
Authorization: Digest username="admin", realm="me@kennethreitz.com", nonce="8dc5e7974a86a6fcc3cf73230b0c4a93", uri="/digest-auth/auth/admin/123456", response="9955ac79f6a51a876a326449447f549d", qop=auth, nc=00000002, cnonce="2fa61501d47a9d39", algorithm=MD5, opaque="64b7f68b386c3acc38131f7472aa2079"
// 5. Authentication successful, response
HTTP/1.1 200 OK
Date: Fri, 12 Aug 2022 07:11:09 GMT
Content-Type: application/json
Content-Length: 48
Connection: keep-alive
Server: gunicorn/19.9.0
Set-Cookie: fake=fake_value; Path=/
Set-Cookie: stale_after=never; Path=/
Access-Control-Allow-Origin: *
Access-Control-Allow-Credentials: true
{
"authenticated": true,
"user": "admin"
}
|
HttpClient 5 Interceptor
Interceptor in HttpClient 5 can intercept the request process at all stages of processing, through the HttpClientBuilder
in the method about Interceptor
can see the nodes can be intercepted.
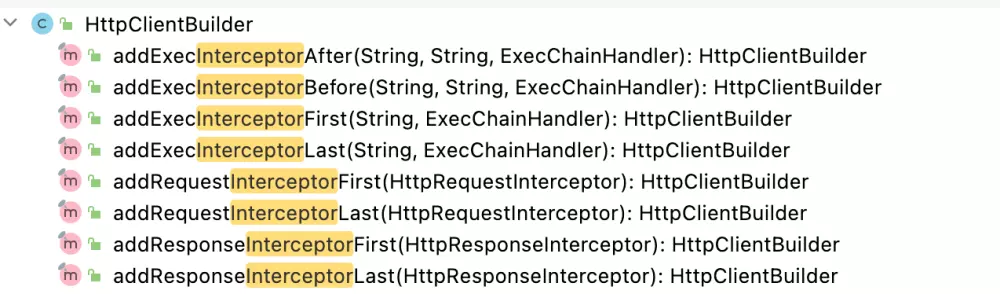
The following example is written to initiate three requests, each with a request-id
parameter in the request header herader, and then end with a 404 response for requests with a request-id
value of 2.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
|
package com.wdbyte.httpclient;
import java.io.IOException;
import java.util.concurrent.atomic.AtomicLong;
import org.apache.hc.client5.http.classic.ExecChain;
import org.apache.hc.client5.http.classic.ExecChain.Scope;
import org.apache.hc.client5.http.classic.ExecChainHandler;
import org.apache.hc.client5.http.classic.methods.HttpGet;
import org.apache.hc.client5.http.impl.ChainElement;
import org.apache.hc.client5.http.impl.classic.CloseableHttpClient;
import org.apache.hc.client5.http.impl.classic.CloseableHttpResponse;
import org.apache.hc.client5.http.impl.classic.HttpClients;
import org.apache.hc.core5.http.ClassicHttpRequest;
import org.apache.hc.core5.http.ClassicHttpResponse;
import org.apache.hc.core5.http.ContentType;
import org.apache.hc.core5.http.EntityDetails;
import org.apache.hc.core5.http.Header;
import org.apache.hc.core5.http.HttpEntity;
import org.apache.hc.core5.http.HttpException;
import org.apache.hc.core5.http.HttpRequest;
import org.apache.hc.core5.http.HttpRequestInterceptor;
import org.apache.hc.core5.http.HttpStatus;
import org.apache.hc.core5.http.io.entity.EntityUtils;
import org.apache.hc.core5.http.io.entity.StringEntity;
import org.apache.hc.core5.http.message.BasicClassicHttpResponse;
import org.apache.hc.core5.http.protocol.HttpContext;
/**
* Demonstrates how to customize processing with interception at request and response time.
*/
public class HttpClient5Interceptors {
public static void main(final String[] args) throws Exception {
try (final CloseableHttpClient httpclient = HttpClients.custom()
// Add a request id to the request header
.addRequestInterceptorFirst(new HttpRequestInterceptor() {
private final AtomicLong count = new AtomicLong(0);
@Override
public void process(
final HttpRequest request,
final EntityDetails entity,
final HttpContext context) throws HttpException, IOException {
request.setHeader("request-id", Long.toString(count.incrementAndGet()));
}
})
.addExecInterceptorAfter(ChainElement.PROTOCOL.name(), "custom", new ExecChainHandler() {
// For request id 2, simulate a 404 response and customize the content of the response.
@Override
public ClassicHttpResponse execute(
final ClassicHttpRequest request,
final Scope scope,
final ExecChain chain) throws IOException, HttpException {
final Header idHeader = request.getFirstHeader("request-id");
if (idHeader != null && "2".equalsIgnoreCase(idHeader.getValue())) {
final ClassicHttpResponse response = new BasicClassicHttpResponse(HttpStatus.SC_NOT_FOUND,
"Oppsie");
response.setEntity(new StringEntity("bad luck", ContentType.TEXT_PLAIN));
return response;
} else {
return chain.proceed(request, scope);
}
}
})
.build()) {
for (int i = 0; i < 3; i++) {
final HttpGet httpget = new HttpGet("http://httpbin.org/get");
try (final CloseableHttpResponse response = httpclient.execute(httpget)) {
System.out.println("----------------------------------------");
System.out.println("Execute request " + httpget.getMethod() + " " + httpget.getUri());
System.out.println(response.getCode() + " " + response.getReasonPhrase());
System.out.println(EntityUtils.toString(response.getEntity()));
}
}
}
}
}
|
The output results are as follows.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
|
----------------------------------------
Execute request GET http://httpbin.org/get
200 OK
{
"args": {},
"headers": {
"Accept-Encoding": "gzip, x-gzip, deflate",
"Host": "httpbin.org",
"Request-Id": "1",
"User-Agent": "Apache-HttpClient/5.1.3 (Java/1.8.0_151)",
"X-Amzn-Trace-Id": "Root=1-62f615ba-658ccd42182d22534dbba82c"
},
"origin": "42.120.75.221",
"url": "http://httpbin.org/get"
}
----------------------------------------
Execute request GET http://httpbin.org/get
404 Oppsie
bad luck
----------------------------------------
Execute request GET http://httpbin.org/get
200 OK
{
"args": {},
"headers": {
"Accept-Encoding": "gzip, x-gzip, deflate",
"Host": "httpbin.org",
"Request-Id": "3",
"User-Agent": "Apache-HttpClient/5.1.3 (Java/1.8.0_151)",
"X-Amzn-Trace-Id": "Root=1-62f615bb-4eb6ba10736ace0e21d0cb8c"
},
"origin": "42.120.75.221",
"url": "http://httpbin.org/get"
}
|
As always, the article code is stored at Github.com/niumoo/javaNotes.
Reference https://www.wdbyte.com/tool/httpclient5.html